Faster normalization of image (numpy array) The Next CEO of Stack OverflowPeak detection in a 2D arrayDump a NumPy array into a csv fileWhy are elementwise additions much faster in separate loops than in a combined loop?Why is it faster to process a sorted array than an unsorted array?Why does Python code run faster in a function?Is < faster than <=?Numpy dot() and array casting performace optimizationDifference between every pair of columns of two numpy arrays (how to do it more efficiently)?Why is [] faster than list()?Faster performance for normalizing a numpy array?
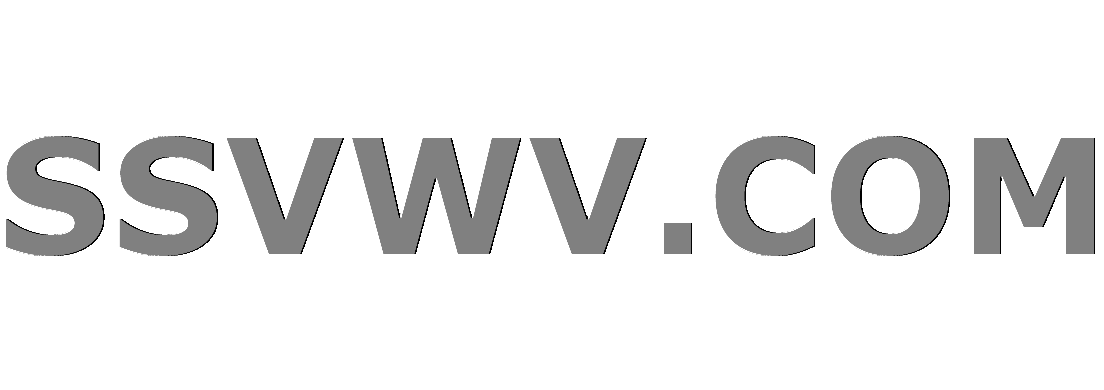
Multi tool use
Customer Requests (Sometimes) Drive Me Bonkers!
How to count occurrences of text in a file?
How do spells that require an ability check vs. the caster's spell save DC work?
Example of a Mathematician/Physicist whose Other Publications during their PhD eclipsed their PhD Thesis
How do scammers retract money, while you can’t?
Visit to the USA with ESTA approved before trip to Iran
What makes a siege story/plot interesting?
Was a professor correct to chastise me for writing "Prof. X" rather than "Professor X"?
Text adventure game code
How to make a variable always equal to the result of some calculations?
How do I solve this limit?
How can I open an app using Terminal?
Can I equip Skullclamp on a creature I am sacrificing?
What is meant by a M next to a roman numeral?
Why here is plural "We went to the movies last night."
Are there languages with no euphemisms?
Science fiction (dystopian) short story set after WWIII
Can a caster that cast Polymorph on themselves stop concentrating at any point even if their Int is low?
Is a stroke of luck acceptable after a series of unfavorable events?
Implement the Thanos sorting algorithm
Why didn't Theresa May consult with Parliament before negotiating a deal with the EU?
Anatomically Correct Strange Women In Ponds Distributing Swords
Only print output after finding pattern
Putting a 2D region plot under a 3D plot
Faster normalization of image (numpy array)
The Next CEO of Stack OverflowPeak detection in a 2D arrayDump a NumPy array into a csv fileWhy are elementwise additions much faster in separate loops than in a combined loop?Why is it faster to process a sorted array than an unsorted array?Why does Python code run faster in a function?Is < faster than <=?Numpy dot() and array casting performace optimizationDifference between every pair of columns of two numpy arrays (how to do it more efficiently)?Why is [] faster than list()?Faster performance for normalizing a numpy array?
I've got function that takes some image as a input and changes it values (scales) in a way that average value will be 96
. Here is the function:
def normalize_image(image: np.ndarray):
image_median = np.median(image[image > 0])
image = image * 96.0 / image_median
image[image > 255] = 255
return image
I am using python 3.5.3 and numpy 1.15.2. I profiled my code with cProfile and it turned out that this function takes 6% of all time (in some scenarios up to 25% of all time) having only 50 calls. These array have shape of (155,256,256).
I am not very experienced with optimizing python and I wonder if this could be made faster somehow?
Normally I would start with using SIMD optimization but numpy use them heavily already.
python performance numpy
add a comment |
I've got function that takes some image as a input and changes it values (scales) in a way that average value will be 96
. Here is the function:
def normalize_image(image: np.ndarray):
image_median = np.median(image[image > 0])
image = image * 96.0 / image_median
image[image > 255] = 255
return image
I am using python 3.5.3 and numpy 1.15.2. I profiled my code with cProfile and it turned out that this function takes 6% of all time (in some scenarios up to 25% of all time) having only 50 calls. These array have shape of (155,256,256).
I am not very experienced with optimizing python and I wonder if this could be made faster somehow?
Normally I would start with using SIMD optimization but numpy use them heavily already.
python performance numpy
1
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
1
Microoptimization: replaceimage = image * 96.0 / image_median
withimage = image * (96.0 / image_median)
.
– Warren Weckesser
Mar 8 at 12:49
add a comment |
I've got function that takes some image as a input and changes it values (scales) in a way that average value will be 96
. Here is the function:
def normalize_image(image: np.ndarray):
image_median = np.median(image[image > 0])
image = image * 96.0 / image_median
image[image > 255] = 255
return image
I am using python 3.5.3 and numpy 1.15.2. I profiled my code with cProfile and it turned out that this function takes 6% of all time (in some scenarios up to 25% of all time) having only 50 calls. These array have shape of (155,256,256).
I am not very experienced with optimizing python and I wonder if this could be made faster somehow?
Normally I would start with using SIMD optimization but numpy use them heavily already.
python performance numpy
I've got function that takes some image as a input and changes it values (scales) in a way that average value will be 96
. Here is the function:
def normalize_image(image: np.ndarray):
image_median = np.median(image[image > 0])
image = image * 96.0 / image_median
image[image > 255] = 255
return image
I am using python 3.5.3 and numpy 1.15.2. I profiled my code with cProfile and it turned out that this function takes 6% of all time (in some scenarios up to 25% of all time) having only 50 calls. These array have shape of (155,256,256).
I am not very experienced with optimizing python and I wonder if this could be made faster somehow?
Normally I would start with using SIMD optimization but numpy use them heavily already.
python performance numpy
python performance numpy
edited Mar 8 at 12:35
wdudzik
asked Mar 8 at 12:25
wdudzikwdudzik
823717
823717
1
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
1
Microoptimization: replaceimage = image * 96.0 / image_median
withimage = image * (96.0 / image_median)
.
– Warren Weckesser
Mar 8 at 12:49
add a comment |
1
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
1
Microoptimization: replaceimage = image * 96.0 / image_median
withimage = image * (96.0 / image_median)
.
– Warren Weckesser
Mar 8 at 12:49
1
1
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
1
1
Microoptimization: replace
image = image * 96.0 / image_median
with image = image * (96.0 / image_median)
.– Warren Weckesser
Mar 8 at 12:49
Microoptimization: replace
image = image * 96.0 / image_median
with image = image * (96.0 / image_median)
.– Warren Weckesser
Mar 8 at 12:49
add a comment |
1 Answer
1
active
oldest
votes
There is not much you can do quickly here.
You already use NumPy in a vectorized manner, so internally C code is executed that probably is already quite optimized.
The calculation of the median can take much longer than calculating the mean (because sorting is involved). Consider replacing it.
Adding some parentheses should save a division of the array
image = image * (96.0 / image_median)
because between operators of equal precedence Python goes from left to right.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55063209%2ffaster-normalization-of-image-numpy-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is not much you can do quickly here.
You already use NumPy in a vectorized manner, so internally C code is executed that probably is already quite optimized.
The calculation of the median can take much longer than calculating the mean (because sorting is involved). Consider replacing it.
Adding some parentheses should save a division of the array
image = image * (96.0 / image_median)
because between operators of equal precedence Python goes from left to right.
add a comment |
There is not much you can do quickly here.
You already use NumPy in a vectorized manner, so internally C code is executed that probably is already quite optimized.
The calculation of the median can take much longer than calculating the mean (because sorting is involved). Consider replacing it.
Adding some parentheses should save a division of the array
image = image * (96.0 / image_median)
because between operators of equal precedence Python goes from left to right.
add a comment |
There is not much you can do quickly here.
You already use NumPy in a vectorized manner, so internally C code is executed that probably is already quite optimized.
The calculation of the median can take much longer than calculating the mean (because sorting is involved). Consider replacing it.
Adding some parentheses should save a division of the array
image = image * (96.0 / image_median)
because between operators of equal precedence Python goes from left to right.
There is not much you can do quickly here.
You already use NumPy in a vectorized manner, so internally C code is executed that probably is already quite optimized.
The calculation of the median can take much longer than calculating the mean (because sorting is involved). Consider replacing it.
Adding some parentheses should save a division of the array
image = image * (96.0 / image_median)
because between operators of equal precedence Python goes from left to right.
answered Mar 8 at 12:50
TrilarionTrilarion
6,84054179
6,84054179
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55063209%2ffaster-normalization-of-image-numpy-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OEto0xHhJWX,Jvd qPVsb1GJeZnoO5sgEXDIcEUxr8 C9z yVP
1
The median calculation could take a long time (needs sorting of the array). Can you maybe replace it with the mean?
– Trilarion
Mar 8 at 12:29
@Trilarion it will provide worse results. I am not sure how big is the difference between mean and median for all of the images that I have. I tested it and there is almost no time difference between mean and median in this case.
– wdudzik
Mar 8 at 12:34
1
Microoptimization: replace
image = image * 96.0 / image_median
withimage = image * (96.0 / image_median)
.– Warren Weckesser
Mar 8 at 12:49