constexpr union with POD Struct members using parameter pack expansions2019 Community Moderator ElectionHow to fill array with contents of a template parameter pack?How do I implement “perfect forwarding” in a class template?Compiling with gcc fails if using lambda function for QObject::connect()gcc:g++ being bureaucratic with template template friendsAddition of two matrix using structerror: invalid user defined conversion from char to const key_type&Cannot initialize const int from unpacked tupleHow to return a static const int std::array from a method?Function receiving a const struct and cin commendWhy did this error occur? c++ library buildconstexpr struct member initialisation
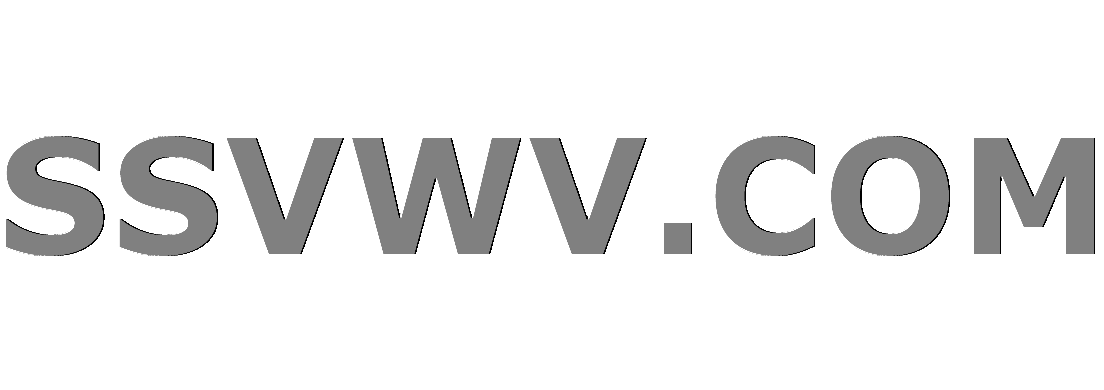
Multi tool use
What is this tube in a jet engine's air intake?
Called into a meeting and told we are being made redundant (laid off) and "not to share outside". Can I tell my partner?
Under what conditions can the right to remain silent be revoked in the USA?
ESPP--any reason not to go all in?
Why do we say 'Pairwise Disjoint', rather than 'Disjoint'?
How exactly does an Ethernet collision happen in the cable, since nodes use different circuits for Tx and Rx?
What is better: yes / no radio, or simple checkbox?
Do Paladin Auras of Differing Oaths Stack?
I can't die. Who am I?
Is divide-by-zero a security vulnerability?
One circle's diameter is different from others within a series of circles
Why does Central Limit Theorem break down in my simulation?
Why is it common in European airports not to display the gate for flights until around 45-90 minutes before departure, unlike other places?
Count each bit-position separately over many 64-bit bitmasks, with AVX but not AVX2
Idiom for feeling after taking risk and someone else being rewarded
How do you make a gun that shoots melee weapons and/or swords?
What can I do if someone tampers with my SSH public key?
If nine coins are tossed, what is the probability that the number of heads is even?
School performs periodic password audits. Is my password compromised?
Why aren't there more Gauls like Obelix?
Help! My Character is too much for her story!
Can I negotiate a patent idea for a raise, under French law?
Can I take the the bonus-action attack from Two-Weapon Fighting without taking the Attack action?
Can the Witch Sight warlock invocation see through the Mirror Image spell?
constexpr union with POD Struct members using parameter pack expansions
2019 Community Moderator ElectionHow to fill array with contents of a template parameter pack?How do I implement “perfect forwarding” in a class template?Compiling with gcc fails if using lambda function for QObject::connect()gcc:g++ being bureaucratic with template template friendsAddition of two matrix using structerror: invalid user defined conversion from char to const key_type&Cannot initialize const int from unpacked tupleHow to return a static const int std::array from a method?Function receiving a const struct and cin commendWhy did this error occur? c++ library buildconstexpr struct member initialisation
I am trying construct a constexpr union which wraps 3 types. The union can contain a raw uint8_t array
or fixed length arrays of PODTypeA
or PODTypeB
. The lengths of these arrays are known at compile time and given by constexpr values.
The POD structures are very simple:
struct PODTypeA
int a;
int b;
;
or
struct PODTypeB
uint32_t a;
uint32_t b;
;
The embedded environment does not have the standard template library available. As such, I cannot use the constexpr std::array<T,N>
which would make things a lot easier.
Shown below is a constexpr Array(const Args&... args)
array implementation, which should function as a constexpr data member to hold array fields.
template <typename T, std::size_t Size>
struct Array
T data[Size];
template <typename ...Args>
constexpr Array(const Args&... args)
: data args...
;
I don't fully understand how the parameter pack expansion works (effectively memcpy(ing) the array via the compiler to the specified union member). If someone could explain that it would be a plus. I found the above Array template parameter pack expander in the checked answer to the following stack overlflow question (where there is also a live example).
I put this in a coliru project but I am having lots of compile issues.
I have 3 constructors for the union, each one of these takes a fixed length array parameter as follows:
constexpr static auto gSizeBytes = 2048;
constexpr static int gArraySizeA = 2;
constexpr static int gArraySizeB = 3;
union UnionStruct
explicit constexpr UnionStruct(
const uint8_t(&rParam)[gSizeBytes] = )
: byteArray(rParam)
explicit constexpr UnionStruct(
const PODTypeA(&rParam)[gArraySizeA])
: arrayA(rParam)
explicit constexpr UnionStruct(
const PODTypeB(&rParam)[gArraySizeB])
: arrayB(rParam)
Array<uint8_t, gSizeBytes> byteArray;
Array<PODTypeA, gArraySizeA> arrayA;
Array<PODTypeB, gArraySizeB> arrayB;
;
And here is how I expect to use it:
int main()
PODTypeA[UnionStruct::gArraySizeA] arrayTypeA = 1,2, 3,4;
// construct union from fixed PODTypeA array with 2 elements
UnionStruct foo (arrayTypeA);
The compiler issues the following errors:
g++ -std=c++17 -O2 -Wall -pedantic -pthread main.cpp && ./a.out
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = unsigned char [2048]; T = unsigned char; long unsigned int Size = 2048]':
main.cpp:34:27: required from here
main.cpp:12:25: error: invalid conversion from 'const unsigned char*' to 'unsigned char' [-fpermissive]
: data args...
^
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeA [2]; T = PODTypeA; long unsigned int Size = 2]':
main.cpp:39:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeA*' to 'int' [-fpermissive]
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeB [3]; T = PODTypeB; long unsigned int Size = 3]':
main.cpp:44:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeB*' to 'uint32_t' aka 'unsigned int' [-fpermissive]
main.cpp: In function 'int main()':
main.cpp:54:14: error: expected identifier before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:14: error: expected ']' before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
]
main.cpp:54:13: error: structured binding declaration cannot have type 'PODTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:13: note: type must be cv-qualified 'auto' or reference to cv-qualified 'auto'
main.cpp:54:13: error: empty structured binding declaration
main.cpp:54:17: error: expected initializer before 'arrayTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^~~~~~~~~~
main.cpp:56:22: error: 'arrayTypeA' was not declared in this scope
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
main.cpp:56:22: note: suggested alternative: 'gArraySizeA'
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
gArraySizeA
main.cpp:54:13: warning: unused structured binding declaration [-Wunused-variable]
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
c++11 union c++17 constexpr
add a comment |
I am trying construct a constexpr union which wraps 3 types. The union can contain a raw uint8_t array
or fixed length arrays of PODTypeA
or PODTypeB
. The lengths of these arrays are known at compile time and given by constexpr values.
The POD structures are very simple:
struct PODTypeA
int a;
int b;
;
or
struct PODTypeB
uint32_t a;
uint32_t b;
;
The embedded environment does not have the standard template library available. As such, I cannot use the constexpr std::array<T,N>
which would make things a lot easier.
Shown below is a constexpr Array(const Args&... args)
array implementation, which should function as a constexpr data member to hold array fields.
template <typename T, std::size_t Size>
struct Array
T data[Size];
template <typename ...Args>
constexpr Array(const Args&... args)
: data args...
;
I don't fully understand how the parameter pack expansion works (effectively memcpy(ing) the array via the compiler to the specified union member). If someone could explain that it would be a plus. I found the above Array template parameter pack expander in the checked answer to the following stack overlflow question (where there is also a live example).
I put this in a coliru project but I am having lots of compile issues.
I have 3 constructors for the union, each one of these takes a fixed length array parameter as follows:
constexpr static auto gSizeBytes = 2048;
constexpr static int gArraySizeA = 2;
constexpr static int gArraySizeB = 3;
union UnionStruct
explicit constexpr UnionStruct(
const uint8_t(&rParam)[gSizeBytes] = )
: byteArray(rParam)
explicit constexpr UnionStruct(
const PODTypeA(&rParam)[gArraySizeA])
: arrayA(rParam)
explicit constexpr UnionStruct(
const PODTypeB(&rParam)[gArraySizeB])
: arrayB(rParam)
Array<uint8_t, gSizeBytes> byteArray;
Array<PODTypeA, gArraySizeA> arrayA;
Array<PODTypeB, gArraySizeB> arrayB;
;
And here is how I expect to use it:
int main()
PODTypeA[UnionStruct::gArraySizeA] arrayTypeA = 1,2, 3,4;
// construct union from fixed PODTypeA array with 2 elements
UnionStruct foo (arrayTypeA);
The compiler issues the following errors:
g++ -std=c++17 -O2 -Wall -pedantic -pthread main.cpp && ./a.out
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = unsigned char [2048]; T = unsigned char; long unsigned int Size = 2048]':
main.cpp:34:27: required from here
main.cpp:12:25: error: invalid conversion from 'const unsigned char*' to 'unsigned char' [-fpermissive]
: data args...
^
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeA [2]; T = PODTypeA; long unsigned int Size = 2]':
main.cpp:39:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeA*' to 'int' [-fpermissive]
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeB [3]; T = PODTypeB; long unsigned int Size = 3]':
main.cpp:44:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeB*' to 'uint32_t' aka 'unsigned int' [-fpermissive]
main.cpp: In function 'int main()':
main.cpp:54:14: error: expected identifier before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:14: error: expected ']' before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
]
main.cpp:54:13: error: structured binding declaration cannot have type 'PODTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:13: note: type must be cv-qualified 'auto' or reference to cv-qualified 'auto'
main.cpp:54:13: error: empty structured binding declaration
main.cpp:54:17: error: expected initializer before 'arrayTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^~~~~~~~~~
main.cpp:56:22: error: 'arrayTypeA' was not declared in this scope
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
main.cpp:56:22: note: suggested alternative: 'gArraySizeA'
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
gArraySizeA
main.cpp:54:13: warning: unused structured binding declaration [-Wunused-variable]
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
c++11 union c++17 constexpr
IntbyteArray(rParam)
, you are attempting to initialiizebyteArray.data[0]
withrParam
. The former isunsigned char
, the latter isunsigned char*
. Hence the first error.
– Igor Tandetnik
Mar 7 at 4:33
Array declaration goes like this:PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.
– Igor Tandetnik
Mar 7 at 4:38
add a comment |
I am trying construct a constexpr union which wraps 3 types. The union can contain a raw uint8_t array
or fixed length arrays of PODTypeA
or PODTypeB
. The lengths of these arrays are known at compile time and given by constexpr values.
The POD structures are very simple:
struct PODTypeA
int a;
int b;
;
or
struct PODTypeB
uint32_t a;
uint32_t b;
;
The embedded environment does not have the standard template library available. As such, I cannot use the constexpr std::array<T,N>
which would make things a lot easier.
Shown below is a constexpr Array(const Args&... args)
array implementation, which should function as a constexpr data member to hold array fields.
template <typename T, std::size_t Size>
struct Array
T data[Size];
template <typename ...Args>
constexpr Array(const Args&... args)
: data args...
;
I don't fully understand how the parameter pack expansion works (effectively memcpy(ing) the array via the compiler to the specified union member). If someone could explain that it would be a plus. I found the above Array template parameter pack expander in the checked answer to the following stack overlflow question (where there is also a live example).
I put this in a coliru project but I am having lots of compile issues.
I have 3 constructors for the union, each one of these takes a fixed length array parameter as follows:
constexpr static auto gSizeBytes = 2048;
constexpr static int gArraySizeA = 2;
constexpr static int gArraySizeB = 3;
union UnionStruct
explicit constexpr UnionStruct(
const uint8_t(&rParam)[gSizeBytes] = )
: byteArray(rParam)
explicit constexpr UnionStruct(
const PODTypeA(&rParam)[gArraySizeA])
: arrayA(rParam)
explicit constexpr UnionStruct(
const PODTypeB(&rParam)[gArraySizeB])
: arrayB(rParam)
Array<uint8_t, gSizeBytes> byteArray;
Array<PODTypeA, gArraySizeA> arrayA;
Array<PODTypeB, gArraySizeB> arrayB;
;
And here is how I expect to use it:
int main()
PODTypeA[UnionStruct::gArraySizeA] arrayTypeA = 1,2, 3,4;
// construct union from fixed PODTypeA array with 2 elements
UnionStruct foo (arrayTypeA);
The compiler issues the following errors:
g++ -std=c++17 -O2 -Wall -pedantic -pthread main.cpp && ./a.out
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = unsigned char [2048]; T = unsigned char; long unsigned int Size = 2048]':
main.cpp:34:27: required from here
main.cpp:12:25: error: invalid conversion from 'const unsigned char*' to 'unsigned char' [-fpermissive]
: data args...
^
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeA [2]; T = PODTypeA; long unsigned int Size = 2]':
main.cpp:39:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeA*' to 'int' [-fpermissive]
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeB [3]; T = PODTypeB; long unsigned int Size = 3]':
main.cpp:44:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeB*' to 'uint32_t' aka 'unsigned int' [-fpermissive]
main.cpp: In function 'int main()':
main.cpp:54:14: error: expected identifier before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:14: error: expected ']' before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
]
main.cpp:54:13: error: structured binding declaration cannot have type 'PODTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:13: note: type must be cv-qualified 'auto' or reference to cv-qualified 'auto'
main.cpp:54:13: error: empty structured binding declaration
main.cpp:54:17: error: expected initializer before 'arrayTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^~~~~~~~~~
main.cpp:56:22: error: 'arrayTypeA' was not declared in this scope
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
main.cpp:56:22: note: suggested alternative: 'gArraySizeA'
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
gArraySizeA
main.cpp:54:13: warning: unused structured binding declaration [-Wunused-variable]
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
c++11 union c++17 constexpr
I am trying construct a constexpr union which wraps 3 types. The union can contain a raw uint8_t array
or fixed length arrays of PODTypeA
or PODTypeB
. The lengths of these arrays are known at compile time and given by constexpr values.
The POD structures are very simple:
struct PODTypeA
int a;
int b;
;
or
struct PODTypeB
uint32_t a;
uint32_t b;
;
The embedded environment does not have the standard template library available. As such, I cannot use the constexpr std::array<T,N>
which would make things a lot easier.
Shown below is a constexpr Array(const Args&... args)
array implementation, which should function as a constexpr data member to hold array fields.
template <typename T, std::size_t Size>
struct Array
T data[Size];
template <typename ...Args>
constexpr Array(const Args&... args)
: data args...
;
I don't fully understand how the parameter pack expansion works (effectively memcpy(ing) the array via the compiler to the specified union member). If someone could explain that it would be a plus. I found the above Array template parameter pack expander in the checked answer to the following stack overlflow question (where there is also a live example).
I put this in a coliru project but I am having lots of compile issues.
I have 3 constructors for the union, each one of these takes a fixed length array parameter as follows:
constexpr static auto gSizeBytes = 2048;
constexpr static int gArraySizeA = 2;
constexpr static int gArraySizeB = 3;
union UnionStruct
explicit constexpr UnionStruct(
const uint8_t(&rParam)[gSizeBytes] = )
: byteArray(rParam)
explicit constexpr UnionStruct(
const PODTypeA(&rParam)[gArraySizeA])
: arrayA(rParam)
explicit constexpr UnionStruct(
const PODTypeB(&rParam)[gArraySizeB])
: arrayB(rParam)
Array<uint8_t, gSizeBytes> byteArray;
Array<PODTypeA, gArraySizeA> arrayA;
Array<PODTypeB, gArraySizeB> arrayB;
;
And here is how I expect to use it:
int main()
PODTypeA[UnionStruct::gArraySizeA] arrayTypeA = 1,2, 3,4;
// construct union from fixed PODTypeA array with 2 elements
UnionStruct foo (arrayTypeA);
The compiler issues the following errors:
g++ -std=c++17 -O2 -Wall -pedantic -pthread main.cpp && ./a.out
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = unsigned char [2048]; T = unsigned char; long unsigned int Size = 2048]':
main.cpp:34:27: required from here
main.cpp:12:25: error: invalid conversion from 'const unsigned char*' to 'unsigned char' [-fpermissive]
: data args...
^
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeA [2]; T = PODTypeA; long unsigned int Size = 2]':
main.cpp:39:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeA*' to 'int' [-fpermissive]
main.cpp: In instantiation of 'constexpr Array<T, Size>::Array(const Args& ...) [with Args = PODTypeB [3]; T = PODTypeB; long unsigned int Size = 3]':
main.cpp:44:24: required from here
main.cpp:12:25: error: invalid conversion from 'const PODTypeB*' to 'uint32_t' aka 'unsigned int' [-fpermissive]
main.cpp: In function 'int main()':
main.cpp:54:14: error: expected identifier before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:14: error: expected ']' before numeric constant
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
]
main.cpp:54:13: error: structured binding declaration cannot have type 'PODTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
main.cpp:54:13: note: type must be cv-qualified 'auto' or reference to cv-qualified 'auto'
main.cpp:54:13: error: empty structured binding declaration
main.cpp:54:17: error: expected initializer before 'arrayTypeA'
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^~~~~~~~~~
main.cpp:56:22: error: 'arrayTypeA' was not declared in this scope
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
main.cpp:56:22: note: suggested alternative: 'gArraySizeA'
UnionStruct foo (arrayTypeA);
^~~~~~~~~~
gArraySizeA
main.cpp:54:13: warning: unused structured binding declaration [-Wunused-variable]
PODTypeA[2] arrayTypeA = 1,2, 3,4;
^
c++11 union c++17 constexpr
c++11 union c++17 constexpr
edited Mar 7 at 1:28
johnco3
asked Mar 6 at 23:38
johnco3johnco3
92921736
92921736
IntbyteArray(rParam)
, you are attempting to initialiizebyteArray.data[0]
withrParam
. The former isunsigned char
, the latter isunsigned char*
. Hence the first error.
– Igor Tandetnik
Mar 7 at 4:33
Array declaration goes like this:PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.
– Igor Tandetnik
Mar 7 at 4:38
add a comment |
IntbyteArray(rParam)
, you are attempting to initialiizebyteArray.data[0]
withrParam
. The former isunsigned char
, the latter isunsigned char*
. Hence the first error.
– Igor Tandetnik
Mar 7 at 4:33
Array declaration goes like this:PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.
– Igor Tandetnik
Mar 7 at 4:38
Int
byteArray(rParam)
, you are attempting to initialiize byteArray.data[0]
with rParam
. The former is unsigned char
, the latter is unsigned char*
. Hence the first error.– Igor Tandetnik
Mar 7 at 4:33
Int
byteArray(rParam)
, you are attempting to initialiize byteArray.data[0]
with rParam
. The former is unsigned char
, the latter is unsigned char*
. Hence the first error.– Igor Tandetnik
Mar 7 at 4:33
Array declaration goes like this:
PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.– Igor Tandetnik
Mar 7 at 4:38
Array declaration goes like this:
PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.– Igor Tandetnik
Mar 7 at 4:38
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55033896%2fconstexpr-union-with-pod-struct-members-using-parameter-pack-expansions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55033896%2fconstexpr-union-with-pod-struct-members-using-parameter-pack-expansions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jw3Z3JT,J4CxiWe104msjOlRagfBf MVaijXdApjP5H c,HF rcju84OdusdP Vw8VB
Int
byteArray(rParam)
, you are attempting to initialiizebyteArray.data[0]
withrParam
. The former isunsigned char
, the latter isunsigned char*
. Hence the first error.– Igor Tandetnik
Mar 7 at 4:33
Array declaration goes like this:
PODTypeA arrayTypeA[UnionStruct::gArraySizeA]
. You have the bound in the wrong place.– Igor Tandetnik
Mar 7 at 4:38