Python multiple functions with the same decorator execute in main The Next CEO of Stack OverflowWhat is the naming convention in Python for variable and function names?What is the Python equivalent of static variables inside a function?How to return multiple values from a function?What are some common uses for Python decorators?mkdir -p functionality in PythonHow do I detect whether a Python variable is a function?What is a clean, pythonic way to have multiple constructors in Python?How to make a chain of function decorators?How do I get time of a Python program's execution?Why does Python code run faster in a function?
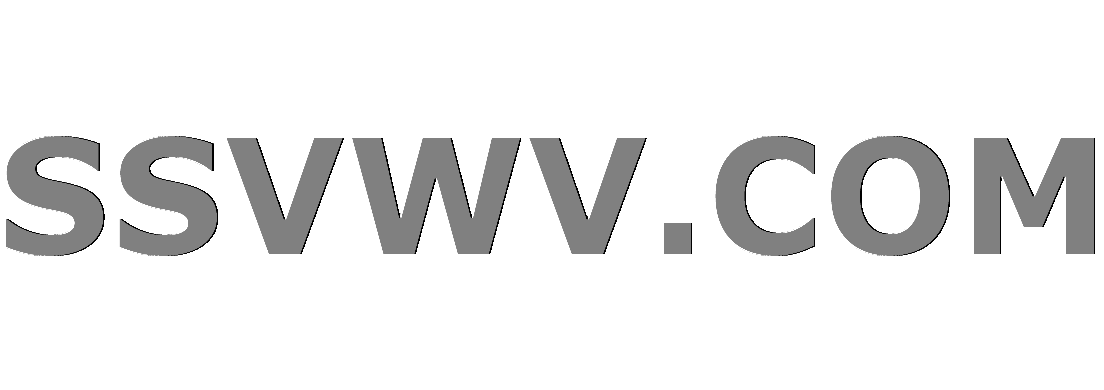
Multi tool use
Reshaping json / reparing json inside shell script (remove trailing comma)
Is there a way to save my career from absolute disaster?
Ising model simulation
Physiological effects of huge anime eyes
Small nick on power cord from an electric alarm clock, and copper wiring exposed but intact
Is it convenient to ask the journal's editor for two additional days to complete a review?
Won the lottery - how do I keep the money?
How do I fit a non linear curve?
How did Beeri the Hittite come up with naming his daughter Yehudit?
What would be the main consequences for a country leaving the WTO?
In the "Harry Potter and the Order of the Phoenix" video game, what potion is used to sabotage Umbridge's speakers?
What is the process for purifying your home if you believe it may have been previously used for pagan worship?
The Ultimate Number Sequence Puzzle
Why did early computer designers eschew integers?
How to use ReplaceAll on an expression that contains a rule
Is a distribution that is normal, but highly skewed, considered Gaussian?
What flight has the highest ratio of timezone difference to flight time?
Calculate the Mean mean of two numbers
Is fine stranded wire ok for main supply line?
Does the Idaho Potato Commission associate potato skins with healthy eating?
Is it correct to say moon starry nights?
Audio Conversion With ADS1243
Is there a reasonable and studied concept of reduction between regular languages?
Raspberry pi 3 B with Ubuntu 18.04 server arm64: what chip
Python multiple functions with the same decorator execute in main
The Next CEO of Stack OverflowWhat is the naming convention in Python for variable and function names?What is the Python equivalent of static variables inside a function?How to return multiple values from a function?What are some common uses for Python decorators?mkdir -p functionality in PythonHow do I detect whether a Python variable is a function?What is a clean, pythonic way to have multiple constructors in Python?How to make a chain of function decorators?How do I get time of a Python program's execution?Why does Python code run faster in a function?
I created a decorator to walk through directories for several functions to do some file operations. Every time when more than one functions with the decorator in the script, only the first one will execute.
import os
import re
import sys
def oswalk_deco(func):
def wrapper(filename, *args):
subdirs = [os.path.abspath(x[0]) for x in os.walk(target_dir)]
subdirs.remove(os.path.abspath(target_dir))
for dir in subdirs:
os.chdir(dir)
for item in os.listdir('.'):
p = re.match(filename, item)
if isinstance(p, re.Match):
match = p.group()
func(match, *args)
return wrapper
def str2uni(string):
if isinstance(string, str):
return string.encode('utf8').decode('unicode_escape')
else:
print('Function "str2uni(string)" only accept strings.')
exit()
@oswalk_deco
def sub_string(filename, regex, substr):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(regex, substr, content)
file.write(content)
@oswalk_deco
def regex_print(filename, string):
with open(filename, 'r') as file:
content = file.read()
relist = re.findall(string, content)
if filename[0] == 'u':
print(str2uni(f'\ufilename[1:-4]'): relist)
elif isinstance(re.match(r'coded2-u.+', filename), re.Match):
print(str2uni(f'\re.search("u[0-9a-z]4,5", filename).group()'): relist)
@oswalk_deco
def docname_format(filename):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(r'docname=".*"', f'docname="filename"', content)
file.write(content)
if __name__ == '__main__':
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
regex_print('.*.svg', 'docname=".*"')
regex_print('.*.svg', 'stroke:none')
sub_string('.*.svg', 'docname=".*"', 'docname="stackoverflow.svg')
It seems like I've missed some important properties in Python?
python decorator
add a comment |
I created a decorator to walk through directories for several functions to do some file operations. Every time when more than one functions with the decorator in the script, only the first one will execute.
import os
import re
import sys
def oswalk_deco(func):
def wrapper(filename, *args):
subdirs = [os.path.abspath(x[0]) for x in os.walk(target_dir)]
subdirs.remove(os.path.abspath(target_dir))
for dir in subdirs:
os.chdir(dir)
for item in os.listdir('.'):
p = re.match(filename, item)
if isinstance(p, re.Match):
match = p.group()
func(match, *args)
return wrapper
def str2uni(string):
if isinstance(string, str):
return string.encode('utf8').decode('unicode_escape')
else:
print('Function "str2uni(string)" only accept strings.')
exit()
@oswalk_deco
def sub_string(filename, regex, substr):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(regex, substr, content)
file.write(content)
@oswalk_deco
def regex_print(filename, string):
with open(filename, 'r') as file:
content = file.read()
relist = re.findall(string, content)
if filename[0] == 'u':
print(str2uni(f'\ufilename[1:-4]'): relist)
elif isinstance(re.match(r'coded2-u.+', filename), re.Match):
print(str2uni(f'\re.search("u[0-9a-z]4,5", filename).group()'): relist)
@oswalk_deco
def docname_format(filename):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(r'docname=".*"', f'docname="filename"', content)
file.write(content)
if __name__ == '__main__':
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
regex_print('.*.svg', 'docname=".*"')
regex_print('.*.svg', 'stroke:none')
sub_string('.*.svg', 'docname=".*"', 'docname="stackoverflow.svg')
It seems like I've missed some important properties in Python?
python decorator
2
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", andsubdirs
is ["foo", "bar"], then in the first iterationchdir(dir)
will try to navigate to C:foo. Then in the second iteration,chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.
– Kevin
Mar 8 at 17:47
... But unless your program is crashing with aFileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.
– Kevin
Mar 8 at 17:49
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07
add a comment |
I created a decorator to walk through directories for several functions to do some file operations. Every time when more than one functions with the decorator in the script, only the first one will execute.
import os
import re
import sys
def oswalk_deco(func):
def wrapper(filename, *args):
subdirs = [os.path.abspath(x[0]) for x in os.walk(target_dir)]
subdirs.remove(os.path.abspath(target_dir))
for dir in subdirs:
os.chdir(dir)
for item in os.listdir('.'):
p = re.match(filename, item)
if isinstance(p, re.Match):
match = p.group()
func(match, *args)
return wrapper
def str2uni(string):
if isinstance(string, str):
return string.encode('utf8').decode('unicode_escape')
else:
print('Function "str2uni(string)" only accept strings.')
exit()
@oswalk_deco
def sub_string(filename, regex, substr):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(regex, substr, content)
file.write(content)
@oswalk_deco
def regex_print(filename, string):
with open(filename, 'r') as file:
content = file.read()
relist = re.findall(string, content)
if filename[0] == 'u':
print(str2uni(f'\ufilename[1:-4]'): relist)
elif isinstance(re.match(r'coded2-u.+', filename), re.Match):
print(str2uni(f'\re.search("u[0-9a-z]4,5", filename).group()'): relist)
@oswalk_deco
def docname_format(filename):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(r'docname=".*"', f'docname="filename"', content)
file.write(content)
if __name__ == '__main__':
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
regex_print('.*.svg', 'docname=".*"')
regex_print('.*.svg', 'stroke:none')
sub_string('.*.svg', 'docname=".*"', 'docname="stackoverflow.svg')
It seems like I've missed some important properties in Python?
python decorator
I created a decorator to walk through directories for several functions to do some file operations. Every time when more than one functions with the decorator in the script, only the first one will execute.
import os
import re
import sys
def oswalk_deco(func):
def wrapper(filename, *args):
subdirs = [os.path.abspath(x[0]) for x in os.walk(target_dir)]
subdirs.remove(os.path.abspath(target_dir))
for dir in subdirs:
os.chdir(dir)
for item in os.listdir('.'):
p = re.match(filename, item)
if isinstance(p, re.Match):
match = p.group()
func(match, *args)
return wrapper
def str2uni(string):
if isinstance(string, str):
return string.encode('utf8').decode('unicode_escape')
else:
print('Function "str2uni(string)" only accept strings.')
exit()
@oswalk_deco
def sub_string(filename, regex, substr):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(regex, substr, content)
file.write(content)
@oswalk_deco
def regex_print(filename, string):
with open(filename, 'r') as file:
content = file.read()
relist = re.findall(string, content)
if filename[0] == 'u':
print(str2uni(f'\ufilename[1:-4]'): relist)
elif isinstance(re.match(r'coded2-u.+', filename), re.Match):
print(str2uni(f'\re.search("u[0-9a-z]4,5", filename).group()'): relist)
@oswalk_deco
def docname_format(filename):
with open(filename, 'r') as file:
content = file.read()
with open(filename, 'w') as file:
content = re.sub(r'docname=".*"', f'docname="filename"', content)
file.write(content)
if __name__ == '__main__':
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
regex_print('.*.svg', 'docname=".*"')
regex_print('.*.svg', 'stroke:none')
sub_string('.*.svg', 'docname=".*"', 'docname="stackoverflow.svg')
It seems like I've missed some important properties in Python?
python decorator
python decorator
edited Mar 8 at 18:17
double-beep
3,08141432
3,08141432
asked Mar 8 at 17:37
ElmruElmru
155
155
2
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", andsubdirs
is ["foo", "bar"], then in the first iterationchdir(dir)
will try to navigate to C:foo. Then in the second iteration,chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.
– Kevin
Mar 8 at 17:47
... But unless your program is crashing with aFileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.
– Kevin
Mar 8 at 17:49
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07
add a comment |
2
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", andsubdirs
is ["foo", "bar"], then in the first iterationchdir(dir)
will try to navigate to C:foo. Then in the second iteration,chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.
– Kevin
Mar 8 at 17:47
... But unless your program is crashing with aFileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.
– Kevin
Mar 8 at 17:49
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07
2
2
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", and subdirs
is ["foo", "bar"], then in the first iteration chdir(dir)
will try to navigate to C:foo. Then in the second iteration, chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.– Kevin
Mar 8 at 17:47
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", and subdirs
is ["foo", "bar"], then in the first iteration chdir(dir)
will try to navigate to C:foo. Then in the second iteration, chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.– Kevin
Mar 8 at 17:47
... But unless your program is crashing with a
FileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.– Kevin
Mar 8 at 17:49
... But unless your program is crashing with a
FileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.– Kevin
Mar 8 at 17:49
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07
add a comment |
1 Answer
1
active
oldest
votes
Your target_dir
defaults to .
, the current working directory, if there's no command line argument given, and in your wrapper
function, the os.walk
function is always called with target_dir
, which, after the os.chdir
call, would refer to one of the subfolders of the first call to the decorated function, so os.walk
naturally would not be able to find any more subfolders under .
, which is already a subfolder.
You can fix this by getting the absolute path of target_dir
first:
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
target_dir = os.path.abspath(target_dir)
add a comment |
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55068298%2fpython-multiple-functions-with-the-same-decorator-execute-in-main%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your target_dir
defaults to .
, the current working directory, if there's no command line argument given, and in your wrapper
function, the os.walk
function is always called with target_dir
, which, after the os.chdir
call, would refer to one of the subfolders of the first call to the decorated function, so os.walk
naturally would not be able to find any more subfolders under .
, which is already a subfolder.
You can fix this by getting the absolute path of target_dir
first:
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
target_dir = os.path.abspath(target_dir)
add a comment |
Your target_dir
defaults to .
, the current working directory, if there's no command line argument given, and in your wrapper
function, the os.walk
function is always called with target_dir
, which, after the os.chdir
call, would refer to one of the subfolders of the first call to the decorated function, so os.walk
naturally would not be able to find any more subfolders under .
, which is already a subfolder.
You can fix this by getting the absolute path of target_dir
first:
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
target_dir = os.path.abspath(target_dir)
add a comment |
Your target_dir
defaults to .
, the current working directory, if there's no command line argument given, and in your wrapper
function, the os.walk
function is always called with target_dir
, which, after the os.chdir
call, would refer to one of the subfolders of the first call to the decorated function, so os.walk
naturally would not be able to find any more subfolders under .
, which is already a subfolder.
You can fix this by getting the absolute path of target_dir
first:
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
target_dir = os.path.abspath(target_dir)
Your target_dir
defaults to .
, the current working directory, if there's no command line argument given, and in your wrapper
function, the os.walk
function is always called with target_dir
, which, after the os.chdir
call, would refer to one of the subfolders of the first call to the decorated function, so os.walk
naturally would not be able to find any more subfolders under .
, which is already a subfolder.
You can fix this by getting the absolute path of target_dir
first:
if len(sys.argv) == 1:
target_dir = '.'
else:
target_dir = sys.argv[1]
target_dir = os.path.abspath(target_dir)
answered Mar 8 at 18:07
blhsingblhsing
41.3k41743
41.3k41743
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55068298%2fpython-multiple-functions-with-the-same-decorator-execute-in-main%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uObJRs fmdg6dv06B1DVa,AI6 1G4S,VgkJ,JzCNVwUcRmS6hpMYRLNax 8L1s,W2eX4M9TwWMl 1ju
2
for dir in subdirs: os.chdir(dir)
looks suspicious to me. If your initial current working directory is "C:", andsubdirs
is ["foo", "bar"], then in the first iterationchdir(dir)
will try to navigate to C:foo. Then in the second iteration,chdir(dir)
will try to navigate to C:foobar instead of C:bar. You need to chdir back up to your original current working directory at the end of each iteration.– Kevin
Mar 8 at 17:47
... But unless your program is crashing with a
FileNotFoundError
, that's probably not the root cause of your problem. Just something to watch out for.– Kevin
Mar 8 at 17:49
Thanks for pointing out the mistake! It IS the reason of this problem and nothing to do with the decorators.
– Elmru
Mar 8 at 18:07