.NET Async/Await - when return task and when await2019 Community Moderator ElectionDifference between decimal, float and double in .NET?How would I run an async Task<T> method synchronously?HttpClient.GetAsync(…) never returns when using await/asyncasync/await - when to return a Task vs void?Using async/await for multiple tasksHow do I return the response from an asynchronous call?How and when to use ‘async’ and ‘await’Async/Await and TasksVisual Basic Async Task instead Sync TaskUsing async/await with a forEach loop
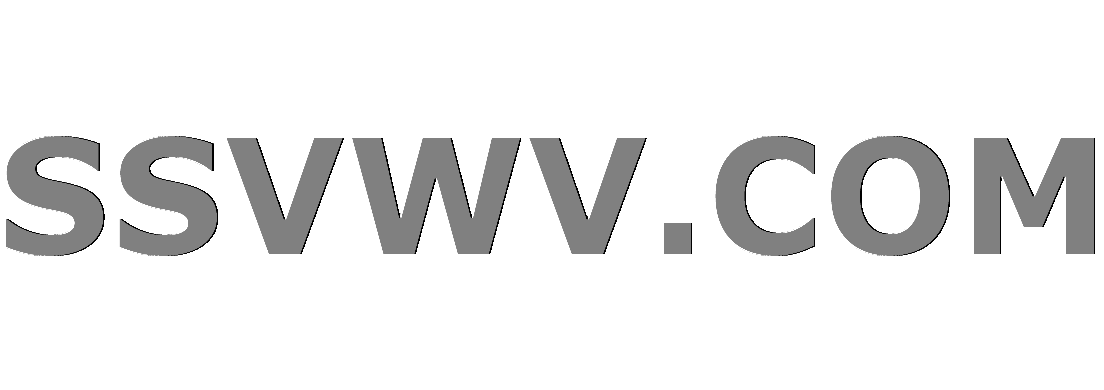
Multi tool use
Why is there is so much iron?
Recruiter wants very extensive technical details about all of my previous work
What is the meaning of まっちろけ?
How to get the n-th line after a grepped one?
Non-trivial topology where only open sets are closed
Relationship between sampajanna definitions in SN 47.2 and SN 47.35
PTIJ: Who should I vote for? (21st Knesset Edition)
How are passwords stolen from companies if they only store hashes?
Print a physical multiplication table
Most cost effective thermostat setting: consistent temperature vs. lowest temperature possible
Does .bashrc contain syntax errors?
How difficult is it to simply disable/disengage the MCAS on Boeing 737 Max 8 & 9 Aircraft?
What is the significance behind "40 days" that often appears in the Bible?
Different outputs for `w`, `who`, `whoami` and `id`
Why is a white electrical wire connected to 2 black wires?
What is the relationship between relativity and the Doppler effect?
How to make healing in an exploration game interesting
What options are left, if Britain cannot decide?
Is there a place to find the pricing for things not mentioned in the PHB? (non-magical)
While on vacation my taxi took a longer route, possibly to scam me out of money. How can I deal with this?
Is a party consisting of only a bard, a cleric, and a warlock functional long-term?
This word with a lot of past tenses
The meaning of 振り in 無茶振り
How could a scammer know the apps on my phone / iTunes account?
.NET Async/Await - when return task and when await
2019 Community Moderator ElectionDifference between decimal, float and double in .NET?How would I run an async Task<T> method synchronously?HttpClient.GetAsync(…) never returns when using await/asyncasync/await - when to return a Task vs void?Using async/await for multiple tasksHow do I return the response from an asynchronous call?How and when to use ‘async’ and ‘await’Async/Await and TasksVisual Basic Async Task instead Sync TaskUsing async/await with a forEach loop
I am in the process of converting synchronous code to async code. I have a windows form application in VB.net which shows the representation of physical devices which are part of a registrationpost being managed by a postmanager.
So the PostManager class is used to manipulate RegistrationPost objects. These registrationpost, contain multiple devices which need to be connected to with Socket communication in one way or another. These devices conform to a IDevice interface by implementing a "connect" sub/function.
Below a condensed version of the code to get an idea of what I'm trying to do:
Private Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, Sub(x) x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
Public Sub ConnectAndStartReading()
Parallel.ForEach(Of IDevice)(AllDevices, Sub(x)
x.Connect()
...
End Sub)
End Sub
Then inside an IDevice:
Public Sub Connect() Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
socket.Connect(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Sub
Ok this is all working but of course I'd like to use async/await to get all the benefits of connecting to an external socket asynchronously. But I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach? See below how this would impact the code example:
' Async/await here as well?
Private async Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
await pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, async Sub(x) await x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Public async Function ConnectAndStartReading() as Task
Parallel.ForEach(Of IDevice)(AllDevices, async Sub(x)
await x.Connect()
... more code, start reading, etc...
End Sub)
End Function
Then inside an IDevice:
Public async Function Connect() as Task Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
await socket.ConnectAsync(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Function
.net vb.net asynchronous async-await
add a comment |
I am in the process of converting synchronous code to async code. I have a windows form application in VB.net which shows the representation of physical devices which are part of a registrationpost being managed by a postmanager.
So the PostManager class is used to manipulate RegistrationPost objects. These registrationpost, contain multiple devices which need to be connected to with Socket communication in one way or another. These devices conform to a IDevice interface by implementing a "connect" sub/function.
Below a condensed version of the code to get an idea of what I'm trying to do:
Private Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, Sub(x) x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
Public Sub ConnectAndStartReading()
Parallel.ForEach(Of IDevice)(AllDevices, Sub(x)
x.Connect()
...
End Sub)
End Sub
Then inside an IDevice:
Public Sub Connect() Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
socket.Connect(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Sub
Ok this is all working but of course I'd like to use async/await to get all the benefits of connecting to an external socket asynchronously. But I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach? See below how this would impact the code example:
' Async/await here as well?
Private async Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
await pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, async Sub(x) await x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Public async Function ConnectAndStartReading() as Task
Parallel.ForEach(Of IDevice)(AllDevices, async Sub(x)
await x.Connect()
... more code, start reading, etc...
End Sub)
End Function
Then inside an IDevice:
Public async Function Connect() as Task Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
await socket.ConnectAsync(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Function
.net vb.net asynchronous async-await
2
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45
add a comment |
I am in the process of converting synchronous code to async code. I have a windows form application in VB.net which shows the representation of physical devices which are part of a registrationpost being managed by a postmanager.
So the PostManager class is used to manipulate RegistrationPost objects. These registrationpost, contain multiple devices which need to be connected to with Socket communication in one way or another. These devices conform to a IDevice interface by implementing a "connect" sub/function.
Below a condensed version of the code to get an idea of what I'm trying to do:
Private Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, Sub(x) x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
Public Sub ConnectAndStartReading()
Parallel.ForEach(Of IDevice)(AllDevices, Sub(x)
x.Connect()
...
End Sub)
End Sub
Then inside an IDevice:
Public Sub Connect() Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
socket.Connect(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Sub
Ok this is all working but of course I'd like to use async/await to get all the benefits of connecting to an external socket asynchronously. But I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach? See below how this would impact the code example:
' Async/await here as well?
Private async Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
await pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, async Sub(x) await x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Public async Function ConnectAndStartReading() as Task
Parallel.ForEach(Of IDevice)(AllDevices, async Sub(x)
await x.Connect()
... more code, start reading, etc...
End Sub)
End Function
Then inside an IDevice:
Public async Function Connect() as Task Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
await socket.ConnectAsync(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Function
.net vb.net asynchronous async-await
I am in the process of converting synchronous code to async code. I have a windows form application in VB.net which shows the representation of physical devices which are part of a registrationpost being managed by a postmanager.
So the PostManager class is used to manipulate RegistrationPost objects. These registrationpost, contain multiple devices which need to be connected to with Socket communication in one way or another. These devices conform to a IDevice interface by implementing a "connect" sub/function.
Below a condensed version of the code to get an idea of what I'm trying to do:
Private Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, Sub(x) x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
Public Sub ConnectAndStartReading()
Parallel.ForEach(Of IDevice)(AllDevices, Sub(x)
x.Connect()
...
End Sub)
End Sub
Then inside an IDevice:
Public Sub Connect() Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
socket.Connect(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Sub
Ok this is all working but of course I'd like to use async/await to get all the benefits of connecting to an external socket asynchronously. But I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach? See below how this would impact the code example:
' Async/await here as well?
Private async Sub Form_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Try
...
Dim pm As New PostManager
await pm.ConnectAndStartPosts
...
Catch ex As Exception
...
End Try
End Sub
Then inside the PostManager Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Friend Sub ConnectAndStartPosts()
' The global variable "Posts" is a List of RegistrationPost objects
Parallel.ForEach(Of RegistrationPost)(Posts, async Sub(x) await x.ConnectAndStartReading())
End Sub
Then inside the RegistrationPost Class:
' Leave this a regular 'Sub' or transform to async Function as Task?
Public async Function ConnectAndStartReading() as Task
Parallel.ForEach(Of IDevice)(AllDevices, async Sub(x)
await x.Connect()
... more code, start reading, etc...
End Sub)
End Function
Then inside an IDevice:
Public async Function Connect() as Task Implements IDevice.Connect
' socket is a global variable of the type Socket(SocketType.Stream, ProtocolType.Tcp)
await socket.ConnectAsync(IP, Port)
... more code gets executed (if connected, log a message)
NotifyPropertyChanged("Connected")
End Function
.net vb.net asynchronous async-await
.net vb.net asynchronous async-await
edited Mar 7 at 15:43


Dmitry Pavliv
31.7k126472
31.7k126472
asked Mar 7 at 15:42
DraszDrasz
114
114
2
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45
add a comment |
2
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45
2
2
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45
add a comment |
1 Answer
1
active
oldest
votes
I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
You should go async
all the way. The overhead of async
/await
, while not zero, is completely lost in the noise as soon as you have real I/O going on.
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach?
Yes. Parallel
is intended for CPU-bound code. For asynchronous concurrency, use Task.WhenAll
. I.e., call your new ConnectAsync
method for each device (e.g., using LINQ's Select
) and then do an Await Task.WhenAll
on the resulting tasks. You can do the same thing for registration posts.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55047665%2fnet-async-await-when-return-task-and-when-await%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
You should go async
all the way. The overhead of async
/await
, while not zero, is completely lost in the noise as soon as you have real I/O going on.
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach?
Yes. Parallel
is intended for CPU-bound code. For asynchronous concurrency, use Task.WhenAll
. I.e., call your new ConnectAsync
method for each device (e.g., using LINQ's Select
) and then do an Await Task.WhenAll
on the resulting tasks. You can do the same thing for registration posts.
add a comment |
I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
You should go async
all the way. The overhead of async
/await
, while not zero, is completely lost in the noise as soon as you have real I/O going on.
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach?
Yes. Parallel
is intended for CPU-bound code. For asynchronous concurrency, use Task.WhenAll
. I.e., call your new ConnectAsync
method for each device (e.g., using LINQ's Select
) and then do an Await Task.WhenAll
on the resulting tasks. You can do the same thing for registration posts.
add a comment |
I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
You should go async
all the way. The overhead of async
/await
, while not zero, is completely lost in the noise as soon as you have real I/O going on.
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach?
Yes. Parallel
is intended for CPU-bound code. For asynchronous concurrency, use Task.WhenAll
. I.e., call your new ConnectAsync
method for each device (e.g., using LINQ's Select
) and then do an Await Task.WhenAll
on the resulting tasks. You can do the same thing for registration posts.
I'm having trouble understanding how far to "bubble up" the async/await keywords? I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created, so probably it isn't needed to go all the way up?
You should go async
all the way. The overhead of async
/await
, while not zero, is completely lost in the noise as soon as you have real I/O going on.
Would it also be better to drop the Parallel.Foreach and go for a regular ForEach? Or even another approach?
Yes. Parallel
is intended for CPU-bound code. For asynchronous concurrency, use Task.WhenAll
. I.e., call your new ConnectAsync
method for each device (e.g., using LINQ's Select
) and then do an Await Task.WhenAll
on the resulting tasks. You can do the same thing for registration posts.
answered Mar 7 at 16:22
Stephen ClearyStephen Cleary
286k47480602
286k47480602
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55047665%2fnet-async-await-when-return-task-and-when-await%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xeZUcjw7nrmdHE,l59 VNwTbB
2
"I'm afraid to get into performance problems because every time you use the async/await keyword, a state machine gets created," - unless you're in a really tight loop (i.e. IO read/processing), that won't matter - plus it is a struct, not an object, until the first actual asynchronous thing happens...
– Marc Gravell♦
Mar 7 at 15:45