Eloquent: filter record based on relationEloquent Relation FilteringLaravel Eloquent Eager Loading : Join same table twiceBitwise and condition in a Laravel relation (Eloquent ORM)Obtaining a list of non-linked models, in many_to_many relations, using Laravel EloquentHow can I get records created_by user with roleLaravel. how can i improve this querySort eloquent model with eager loaded relationship in LaravelMutli relationships with Eloquent and LaravelEloquent whereHas is looking for 'user_id' instead of 'id' even though I defined 'id'How to write laravel query with left join and a inner join?
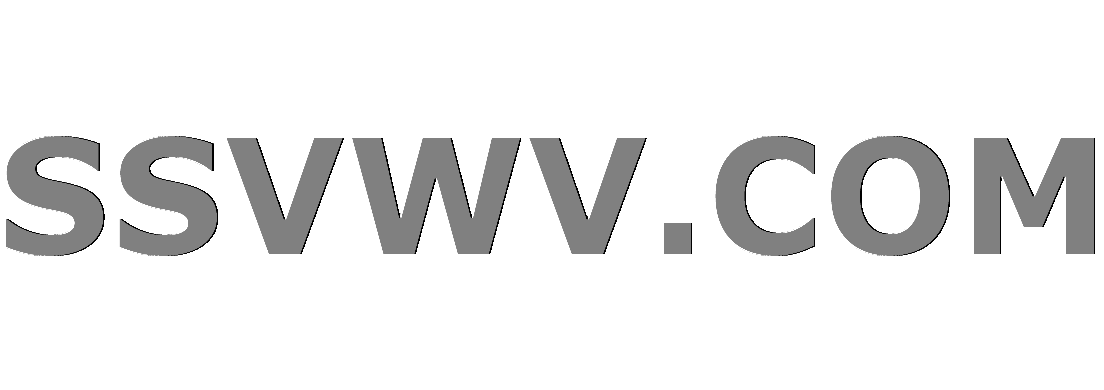
Multi tool use
Teaching indefinite integrals that require special-casing
Why is delta-v is the most useful quantity for planning space travel?
Student evaluations of teaching assistants
Efficiently merge handle parallel feature branches in SFDX
Why does John Bercow say “unlock” after reading out the results of a vote?
Products and sum of cubes in Fibonacci
There is only s̶i̶x̶t̶y one place he can be
How to be diplomatic in refusing to write code that breaches the privacy of our users
Is there a good way to store credentials outside of a password manager?
What will be the benefits of Brexit?
Greatest common substring
Bash method for viewing beginning and end of file
What's the purpose of "true" in bash "if sudo true; then"
At which point does a character regain all their Hit Dice?
What would happen if the UK refused to take part in EU Parliamentary elections?
Is there any reason not to eat food that's been dropped on the surface of the moon?
Understanding "audieritis" in Psalm 94
Why Were Madagascar and New Zealand Discovered So Late?
Was Spock the First Vulcan in Starfleet?
Curses work by shouting - How to avoid collateral damage?
Using parameter substitution on a Bash array
Is HostGator storing my password in plaintext?
when is out of tune ok?
Is a roofing delivery truck likely to crack my driveway slab?
Eloquent: filter record based on relation
Eloquent Relation FilteringLaravel Eloquent Eager Loading : Join same table twiceBitwise and condition in a Laravel relation (Eloquent ORM)Obtaining a list of non-linked models, in many_to_many relations, using Laravel EloquentHow can I get records created_by user with roleLaravel. how can i improve this querySort eloquent model with eager loaded relationship in LaravelMutli relationships with Eloquent and LaravelEloquent whereHas is looking for 'user_id' instead of 'id' even though I defined 'id'How to write laravel query with left join and a inner join?
I'm trying to get customer specific users who don't have an owner role, but it also skips users who don't have any role. Users can have one or multiple roles. I want to get all users either having multiple roles or no role at all, but if the user contains an owner role then only that user should be ignored.
Note: I am using spatie/laravel-permission
which gets users roles from model has roles intermediate table
Here is my scope query
public function scopeForCompany(EloquentBuilder $query, string $customerId): EloquentBuilder
$query->where(function (EloquentBuilder $q)
$q->doesntHave('roles');
$q->orHas('roles');
);
$query->whereHas('roles', function (EloquentBuilder $q)
$q->whereNotIn('name', ['owner']);
);
return $query->where('customer_id', $customerId);;
here is the test
public function it_apply_query_scope_to_get_customer_specific_users_only(): void
$model = new User;
// create non customer users
factory(User::class, 2)->create();
$customer = factory(Customer::class)->create();
foreach (['owner', 'admin', 'user'] as $role)
$role = factory(Role::class)->create(['name' => $role]);
$user = factory(User::class)->create(['customer_id' => $customer->id]);
$user->roles()->save($role);
$scopedUsers = $model->newQuery()->forCompany($customer->id)->get();
$nonScopedUsers = $model->newQuery()->get();
static::assertCount(2, $scopedUsers); // Failed asserting that actual size 0 matches expected size 2.
static::assertCount(5, $nonScopedUsers);
Debug: here is the row query:
"select * from `users` where (not exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?) or exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?)) and exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ? and `name` not in (?)) and `customer_id` = ? and `users`.`deleted_at` is null"
This is what i tried first but didn't worked
return $query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)->where('customer_id', $customerId);
Any help would be appreciated thanks
php mysql laravel eloquent
add a comment |
I'm trying to get customer specific users who don't have an owner role, but it also skips users who don't have any role. Users can have one or multiple roles. I want to get all users either having multiple roles or no role at all, but if the user contains an owner role then only that user should be ignored.
Note: I am using spatie/laravel-permission
which gets users roles from model has roles intermediate table
Here is my scope query
public function scopeForCompany(EloquentBuilder $query, string $customerId): EloquentBuilder
$query->where(function (EloquentBuilder $q)
$q->doesntHave('roles');
$q->orHas('roles');
);
$query->whereHas('roles', function (EloquentBuilder $q)
$q->whereNotIn('name', ['owner']);
);
return $query->where('customer_id', $customerId);;
here is the test
public function it_apply_query_scope_to_get_customer_specific_users_only(): void
$model = new User;
// create non customer users
factory(User::class, 2)->create();
$customer = factory(Customer::class)->create();
foreach (['owner', 'admin', 'user'] as $role)
$role = factory(Role::class)->create(['name' => $role]);
$user = factory(User::class)->create(['customer_id' => $customer->id]);
$user->roles()->save($role);
$scopedUsers = $model->newQuery()->forCompany($customer->id)->get();
$nonScopedUsers = $model->newQuery()->get();
static::assertCount(2, $scopedUsers); // Failed asserting that actual size 0 matches expected size 2.
static::assertCount(5, $nonScopedUsers);
Debug: here is the row query:
"select * from `users` where (not exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?) or exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?)) and exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ? and `name` not in (?)) and `customer_id` = ? and `users`.`deleted_at` is null"
This is what i tried first but didn't worked
return $query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)->where('customer_id', $customerId);
Any help would be appreciated thanks
php mysql laravel eloquent
And what is the error of your code?
– dparoli
Mar 8 at 8:28
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57
add a comment |
I'm trying to get customer specific users who don't have an owner role, but it also skips users who don't have any role. Users can have one or multiple roles. I want to get all users either having multiple roles or no role at all, but if the user contains an owner role then only that user should be ignored.
Note: I am using spatie/laravel-permission
which gets users roles from model has roles intermediate table
Here is my scope query
public function scopeForCompany(EloquentBuilder $query, string $customerId): EloquentBuilder
$query->where(function (EloquentBuilder $q)
$q->doesntHave('roles');
$q->orHas('roles');
);
$query->whereHas('roles', function (EloquentBuilder $q)
$q->whereNotIn('name', ['owner']);
);
return $query->where('customer_id', $customerId);;
here is the test
public function it_apply_query_scope_to_get_customer_specific_users_only(): void
$model = new User;
// create non customer users
factory(User::class, 2)->create();
$customer = factory(Customer::class)->create();
foreach (['owner', 'admin', 'user'] as $role)
$role = factory(Role::class)->create(['name' => $role]);
$user = factory(User::class)->create(['customer_id' => $customer->id]);
$user->roles()->save($role);
$scopedUsers = $model->newQuery()->forCompany($customer->id)->get();
$nonScopedUsers = $model->newQuery()->get();
static::assertCount(2, $scopedUsers); // Failed asserting that actual size 0 matches expected size 2.
static::assertCount(5, $nonScopedUsers);
Debug: here is the row query:
"select * from `users` where (not exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?) or exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?)) and exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ? and `name` not in (?)) and `customer_id` = ? and `users`.`deleted_at` is null"
This is what i tried first but didn't worked
return $query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)->where('customer_id', $customerId);
Any help would be appreciated thanks
php mysql laravel eloquent
I'm trying to get customer specific users who don't have an owner role, but it also skips users who don't have any role. Users can have one or multiple roles. I want to get all users either having multiple roles or no role at all, but if the user contains an owner role then only that user should be ignored.
Note: I am using spatie/laravel-permission
which gets users roles from model has roles intermediate table
Here is my scope query
public function scopeForCompany(EloquentBuilder $query, string $customerId): EloquentBuilder
$query->where(function (EloquentBuilder $q)
$q->doesntHave('roles');
$q->orHas('roles');
);
$query->whereHas('roles', function (EloquentBuilder $q)
$q->whereNotIn('name', ['owner']);
);
return $query->where('customer_id', $customerId);;
here is the test
public function it_apply_query_scope_to_get_customer_specific_users_only(): void
$model = new User;
// create non customer users
factory(User::class, 2)->create();
$customer = factory(Customer::class)->create();
foreach (['owner', 'admin', 'user'] as $role)
$role = factory(Role::class)->create(['name' => $role]);
$user = factory(User::class)->create(['customer_id' => $customer->id]);
$user->roles()->save($role);
$scopedUsers = $model->newQuery()->forCompany($customer->id)->get();
$nonScopedUsers = $model->newQuery()->get();
static::assertCount(2, $scopedUsers); // Failed asserting that actual size 0 matches expected size 2.
static::assertCount(5, $nonScopedUsers);
Debug: here is the row query:
"select * from `users` where (not exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?) or exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ?)) and exists (select * from `roles` inner join `model_has_roles` on `roles`.`id` = `model_has_roles`.`role_id` where `users`.`id` = `model_has_roles`.`model_uuid` and `model_has_roles`.`model_type` = ? and `name` not in (?)) and `customer_id` = ? and `users`.`deleted_at` is null"
This is what i tried first but didn't worked
return $query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)->where('customer_id', $customerId);
Any help would be appreciated thanks
php mysql laravel eloquent
php mysql laravel eloquent
edited Mar 8 at 10:41
ahmed waleed
asked Mar 8 at 8:19
ahmed waleedahmed waleed
505
505
And what is the error of your code?
– dparoli
Mar 8 at 8:28
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57
add a comment |
And what is the error of your code?
– dparoli
Mar 8 at 8:28
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57
And what is the error of your code?
– dparoli
Mar 8 at 8:28
And what is the error of your code?
– dparoli
Mar 8 at 8:28
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.
return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.
return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57
add a comment |
1 Answer
1
active
oldest
votes
You need to do some Or logic for this to happen. I break the query up into 3 pieces.
The statement: "I want to get all users either having multiple roles"
$query->has('roles', '>=', 2);
Next you want all with no roles: "or no role at all".
$query->doesntHave('roles');
And lastly your query correctly filter out where the role cannot be the owner.
$query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
Putting it all together doing something like, with a sub where query. To proper do the Or logic you want.
$query->where(function($builder)
$builder->has('roles', '>=', 2);
$builder->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
);
$builder->orDoesntHave('roles');
In pseudo logical statements this would look something similar to like:
(roles.each.name != 'owner' && count(roles) >= 2) || empty(roles)
Let's see if this help your case, else post the toSql() of the builder and let's figure it out. It's a fairly complex query builder logic this is doing.
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55059196%2feloquent-filter-record-based-on-relation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to do some Or logic for this to happen. I break the query up into 3 pieces.
The statement: "I want to get all users either having multiple roles"
$query->has('roles', '>=', 2);
Next you want all with no roles: "or no role at all".
$query->doesntHave('roles');
And lastly your query correctly filter out where the role cannot be the owner.
$query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
Putting it all together doing something like, with a sub where query. To proper do the Or logic you want.
$query->where(function($builder)
$builder->has('roles', '>=', 2);
$builder->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
);
$builder->orDoesntHave('roles');
In pseudo logical statements this would look something similar to like:
(roles.each.name != 'owner' && count(roles) >= 2) || empty(roles)
Let's see if this help your case, else post the toSql() of the builder and let's figure it out. It's a fairly complex query builder logic this is doing.
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
add a comment |
You need to do some Or logic for this to happen. I break the query up into 3 pieces.
The statement: "I want to get all users either having multiple roles"
$query->has('roles', '>=', 2);
Next you want all with no roles: "or no role at all".
$query->doesntHave('roles');
And lastly your query correctly filter out where the role cannot be the owner.
$query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
Putting it all together doing something like, with a sub where query. To proper do the Or logic you want.
$query->where(function($builder)
$builder->has('roles', '>=', 2);
$builder->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
);
$builder->orDoesntHave('roles');
In pseudo logical statements this would look something similar to like:
(roles.each.name != 'owner' && count(roles) >= 2) || empty(roles)
Let's see if this help your case, else post the toSql() of the builder and let's figure it out. It's a fairly complex query builder logic this is doing.
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
add a comment |
You need to do some Or logic for this to happen. I break the query up into 3 pieces.
The statement: "I want to get all users either having multiple roles"
$query->has('roles', '>=', 2);
Next you want all with no roles: "or no role at all".
$query->doesntHave('roles');
And lastly your query correctly filter out where the role cannot be the owner.
$query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
Putting it all together doing something like, with a sub where query. To proper do the Or logic you want.
$query->where(function($builder)
$builder->has('roles', '>=', 2);
$builder->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
);
$builder->orDoesntHave('roles');
In pseudo logical statements this would look something similar to like:
(roles.each.name != 'owner' && count(roles) >= 2) || empty(roles)
Let's see if this help your case, else post the toSql() of the builder and let's figure it out. It's a fairly complex query builder logic this is doing.
You need to do some Or logic for this to happen. I break the query up into 3 pieces.
The statement: "I want to get all users either having multiple roles"
$query->has('roles', '>=', 2);
Next you want all with no roles: "or no role at all".
$query->doesntHave('roles');
And lastly your query correctly filter out where the role cannot be the owner.
$query->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
Putting it all together doing something like, with a sub where query. To proper do the Or logic you want.
$query->where(function($builder)
$builder->has('roles', '>=', 2);
$builder->whereHas('roles', function (EloquentBuilder $query): void
$query->whereNotIn('name', ['owner']);
)
);
$builder->orDoesntHave('roles');
In pseudo logical statements this would look something similar to like:
(roles.each.name != 'owner' && count(roles) >= 2) || empty(roles)
Let's see if this help your case, else post the toSql() of the builder and let's figure it out. It's a fairly complex query builder logic this is doing.
edited Mar 8 at 9:33
answered Mar 8 at 8:42
Martin HenriksenMartin Henriksen
1,721622
1,721622
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
add a comment |
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I updated the answer there was some wrong logic that would not have passed.
– Martin Henriksen
Mar 8 at 9:33
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
I also update the question please have a look and meanwhile let me try your proposed solution
– ahmed waleed
Mar 8 at 9:40
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
Thanks man your updated solution worked thank you so much.
– ahmed waleed
Mar 8 at 9:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55059196%2feloquent-filter-record-based-on-relation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dH3fhw0Yc,KdSqeO4gVOC,V5Bmewen1p5Sl3i5Xkr2w5,u5gHVjCcVvLYGU8y 6FcGyP LtoQ6bAsnOVZV0XCF7IbnQzHjrClPesY
And what is the error of your code?
– dparoli
Mar 8 at 8:28
Please provide whole query not only a single part I will makes to understand the problem better. anyway try this.
return $query->where(function($query) return $query->whereHas("roles", function($query_2) return $query_2->whereNotIn("name", ["owner"]); ) ->has("roles", ">=", 0); );
– ThataL
Mar 8 at 8:57