How to extract words from a string in JavaHow do I create a Java string from the contents of a file?Extract a specific word from a text in javaWhat is the difference between String and string in C#?Is Java “pass-by-reference” or “pass-by-value”?How to check if a string contains a substring in BashHow do I iterate over the words of a string?How do I read / convert an InputStream into a String in Java?How to check whether a string contains a substring in JavaScript?Does Python have a string 'contains' substring method?How do I check if a string contains a specific word?How do I convert a String to an int in Java?Why is char[] preferred over String for passwords?
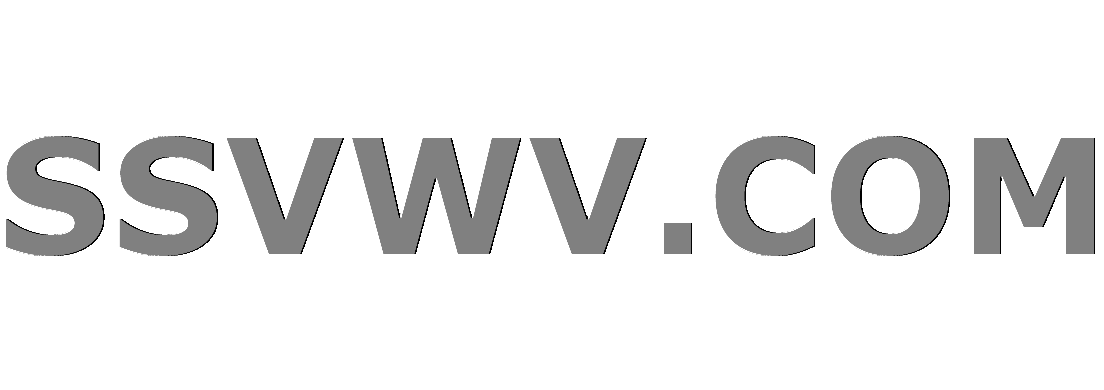
Multi tool use
Identify a stage play about a VR experience in which participants are encouraged to simulate performing horrific activities
Science Fiction story where a man invents a machine that can help him watch history unfold
Is there enough fresh water in the world to eradicate the drinking water crisis?
Can somebody explain Brexit in a few child-proof sentences?
How do I repair my stair bannister?
What was required to accept "troll"?
Golf game boilerplate
Is there an Impartial Brexit Deal comparison site?
Visiting the UK as unmarried couple
Calculating the number of days between 2 dates in Excel
Meta programming: Declare a new struct on the fly
Who must act to prevent Brexit on March 29th?
Why isn't KTEX's runway designation 10/28 instead of 9/27?
Lightning Web Component - do I need to track changes for every single input field in a form
Simulating a probability of 1 of 2^N with less than N random bits
Why are on-board computers allowed to change controls without notifying the pilots?
What is the opposite of 'gravitas'?
Why does this part of the Space Shuttle launch pad seem to be floating in air?
What is the term when two people sing in harmony, but they aren't singing the same notes?
How will losing mobility of one hand affect my career as a programmer?
Does "Dominei" mean something?
Should my PhD thesis be submitted under my legal name?
Can I use my Chinese passport to enter China after I acquired another citizenship?
Have I saved too much for retirement so far?
How to extract words from a string in Java
How do I create a Java string from the contents of a file?Extract a specific word from a text in javaWhat is the difference between String and string in C#?Is Java “pass-by-reference” or “pass-by-value”?How to check if a string contains a substring in BashHow do I iterate over the words of a string?How do I read / convert an InputStream into a String in Java?How to check whether a string contains a substring in JavaScript?Does Python have a string 'contains' substring method?How do I check if a string contains a specific word?How do I convert a String to an int in Java?Why is char[] preferred over String for passwords?
I've imported a file and turned it into a String called readFile. The file contains two lines:
qwertyuiop00%
qwertyuiop
I have already extracted the "00" from the string using:
String number = readFile.substring(11, 13);
I now want to extract the "ert" and the "uio" in "qwertyuiop"
When I try to use the same method as the first, like so:
String e = readFile.substring(16, 19);
String u = readFile.substring(20, 23);
and try to use:
System.out.println(e + "and" + u);
It says string index out of range.
How do I go about this?
Is it because the next two words I want to extract from the string are on the second line?
If so, how do I extract only the second line?
I want to keep it basic, thanks.
UPDATE:
it turns out only the first line of the file is being read, does anyone know how to make it so it reads both lines?
java string substring extract
add a comment |
I've imported a file and turned it into a String called readFile. The file contains two lines:
qwertyuiop00%
qwertyuiop
I have already extracted the "00" from the string using:
String number = readFile.substring(11, 13);
I now want to extract the "ert" and the "uio" in "qwertyuiop"
When I try to use the same method as the first, like so:
String e = readFile.substring(16, 19);
String u = readFile.substring(20, 23);
and try to use:
System.out.println(e + "and" + u);
It says string index out of range.
How do I go about this?
Is it because the next two words I want to extract from the string are on the second line?
If so, how do I extract only the second line?
I want to keep it basic, thanks.
UPDATE:
it turns out only the first line of the file is being read, does anyone know how to make it so it reads both lines?
java string substring extract
It won't work.
What actually happening ?
– Suresh Atta
Oct 22 '14 at 13:37
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18
add a comment |
I've imported a file and turned it into a String called readFile. The file contains two lines:
qwertyuiop00%
qwertyuiop
I have already extracted the "00" from the string using:
String number = readFile.substring(11, 13);
I now want to extract the "ert" and the "uio" in "qwertyuiop"
When I try to use the same method as the first, like so:
String e = readFile.substring(16, 19);
String u = readFile.substring(20, 23);
and try to use:
System.out.println(e + "and" + u);
It says string index out of range.
How do I go about this?
Is it because the next two words I want to extract from the string are on the second line?
If so, how do I extract only the second line?
I want to keep it basic, thanks.
UPDATE:
it turns out only the first line of the file is being read, does anyone know how to make it so it reads both lines?
java string substring extract
I've imported a file and turned it into a String called readFile. The file contains two lines:
qwertyuiop00%
qwertyuiop
I have already extracted the "00" from the string using:
String number = readFile.substring(11, 13);
I now want to extract the "ert" and the "uio" in "qwertyuiop"
When I try to use the same method as the first, like so:
String e = readFile.substring(16, 19);
String u = readFile.substring(20, 23);
and try to use:
System.out.println(e + "and" + u);
It says string index out of range.
How do I go about this?
Is it because the next two words I want to extract from the string are on the second line?
If so, how do I extract only the second line?
I want to keep it basic, thanks.
UPDATE:
it turns out only the first line of the file is being read, does anyone know how to make it so it reads both lines?
java string substring extract
java string substring extract
edited Oct 22 '14 at 14:38
ChatNoir
asked Oct 22 '14 at 13:35
ChatNoirChatNoir
145412
145412
It won't work.
What actually happening ?
– Suresh Atta
Oct 22 '14 at 13:37
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18
add a comment |
It won't work.
What actually happening ?
– Suresh Atta
Oct 22 '14 at 13:37
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18
It won't work.
What actually happening ?– Suresh Atta
Oct 22 '14 at 13:37
It won't work.
What actually happening ?– Suresh Atta
Oct 22 '14 at 13:37
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18
add a comment |
3 Answers
3
active
oldest
votes
If you count the total number of characters for each string, they are more than the indexes your entering.
qwertyuiop00% is 13 characters. Call .length()
method on the string to verify the length is the one you expect.
I would debug with adding the following before:
System.out.println(readFile);
System.out.println(readFile.length());
Note:
qwertyuiop00% qwertyuiop
is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
Note2:
I asked for the parser code since I suspect your using the usual code which is something like:
while ((line = reader.readLine()) != null)
You need to concatenate those lines into one String (though it's not the best approach).
see: How do I create a Java string from the contents of a file?
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
I think your usingreadline()
in your parser, which means your not reading both lines into 1 string.
– Menelaos Bakopoulos
Oct 22 '14 at 13:56
|
show 5 more comments
First split your string into lines, you could do this using
String[] lines = readFile.split("[rn]+");
You may want to read the content directly into a List<String>
using Files.#readAllLines instead.
second, do not use hard coded indexes, use String#indexOf to find them out. If a substring does not occur in your original string, then the method retunrs -1
, always check for that value and call substring
only when the return value is not -1
(0 or greater).
if(lines.length > 1)
int startIndex = lines[1].indexOf("ert");
if(startIndex != -1)
// do what you want
Btw, there is no point in extracting already known substring from a string
System.out.println(e + "and" + u);
is equivalent to
System.out.println("ertanduio");
Knowing the start and end position of a fixed substring makes only sence if you want to do something with rest of original string, for example removing the substrings.
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
add a comment |
You may give this a try:-
Scanner sc=new Scanner(new FileReader(new File(The file path for readFile.txt)));
String st="";
while(sc.hasNext())
st=sc.next();
System.out.println(st.substring(2,5)+" "+"and"+" "+st.substring(6,9));
Check out if it works.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f26508762%2fhow-to-extract-words-from-a-string-in-java%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you count the total number of characters for each string, they are more than the indexes your entering.
qwertyuiop00% is 13 characters. Call .length()
method on the string to verify the length is the one you expect.
I would debug with adding the following before:
System.out.println(readFile);
System.out.println(readFile.length());
Note:
qwertyuiop00% qwertyuiop
is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
Note2:
I asked for the parser code since I suspect your using the usual code which is something like:
while ((line = reader.readLine()) != null)
You need to concatenate those lines into one String (though it's not the best approach).
see: How do I create a Java string from the contents of a file?
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
I think your usingreadline()
in your parser, which means your not reading both lines into 1 string.
– Menelaos Bakopoulos
Oct 22 '14 at 13:56
|
show 5 more comments
If you count the total number of characters for each string, they are more than the indexes your entering.
qwertyuiop00% is 13 characters. Call .length()
method on the string to verify the length is the one you expect.
I would debug with adding the following before:
System.out.println(readFile);
System.out.println(readFile.length());
Note:
qwertyuiop00% qwertyuiop
is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
Note2:
I asked for the parser code since I suspect your using the usual code which is something like:
while ((line = reader.readLine()) != null)
You need to concatenate those lines into one String (though it's not the best approach).
see: How do I create a Java string from the contents of a file?
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
I think your usingreadline()
in your parser, which means your not reading both lines into 1 string.
– Menelaos Bakopoulos
Oct 22 '14 at 13:56
|
show 5 more comments
If you count the total number of characters for each string, they are more than the indexes your entering.
qwertyuiop00% is 13 characters. Call .length()
method on the string to verify the length is the one you expect.
I would debug with adding the following before:
System.out.println(readFile);
System.out.println(readFile.length());
Note:
qwertyuiop00% qwertyuiop
is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
Note2:
I asked for the parser code since I suspect your using the usual code which is something like:
while ((line = reader.readLine()) != null)
You need to concatenate those lines into one String (though it's not the best approach).
see: How do I create a Java string from the contents of a file?
If you count the total number of characters for each string, they are more than the indexes your entering.
qwertyuiop00% is 13 characters. Call .length()
method on the string to verify the length is the one you expect.
I would debug with adding the following before:
System.out.println(readFile);
System.out.println(readFile.length());
Note:
qwertyuiop00% qwertyuiop
is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
Note2:
I asked for the parser code since I suspect your using the usual code which is something like:
while ((line = reader.readLine()) != null)
You need to concatenate those lines into one String (though it's not the best approach).
see: How do I create a Java string from the contents of a file?
edited May 23 '17 at 12:27
Community♦
11
11
answered Oct 22 '14 at 13:42
Menelaos BakopoulosMenelaos Bakopoulos
12.3k846101
12.3k846101
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
I think your usingreadline()
in your parser, which means your not reading both lines into 1 string.
– Menelaos Bakopoulos
Oct 22 '14 at 13:56
|
show 5 more comments
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
I think your usingreadline()
in your parser, which means your not reading both lines into 1 string.
– Menelaos Bakopoulos
Oct 22 '14 at 13:56
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
qwertyuiop00% qwertyuiop all together is 23 characters?
– ChatNoir
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
Can you give us the parsing code? Also have you printed out that this is actually the value within the variable?
– Menelaos Bakopoulos
Oct 22 '14 at 13:45
1
1
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
I think he needs to take the lenght to avoid mistake and also he can test if substring exists : if(string.contains("uio"))
– caps lock
Oct 22 '14 at 13:47
1
1
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
@hheather qwertyuiop00% qwertyuiop is 24 characters since space counts as a character. Unless ofcourse you don't have the space in which it's 23 characters and your indexes are 0 to 22
– Menelaos Bakopoulos
Oct 22 '14 at 13:54
1
1
I think your using
readline()
in your parser, which means your not reading both lines into 1 string.– Menelaos Bakopoulos
Oct 22 '14 at 13:56
I think your using
readline()
in your parser, which means your not reading both lines into 1 string.– Menelaos Bakopoulos
Oct 22 '14 at 13:56
|
show 5 more comments
First split your string into lines, you could do this using
String[] lines = readFile.split("[rn]+");
You may want to read the content directly into a List<String>
using Files.#readAllLines instead.
second, do not use hard coded indexes, use String#indexOf to find them out. If a substring does not occur in your original string, then the method retunrs -1
, always check for that value and call substring
only when the return value is not -1
(0 or greater).
if(lines.length > 1)
int startIndex = lines[1].indexOf("ert");
if(startIndex != -1)
// do what you want
Btw, there is no point in extracting already known substring from a string
System.out.println(e + "and" + u);
is equivalent to
System.out.println("ertanduio");
Knowing the start and end position of a fixed substring makes only sence if you want to do something with rest of original string, for example removing the substrings.
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
add a comment |
First split your string into lines, you could do this using
String[] lines = readFile.split("[rn]+");
You may want to read the content directly into a List<String>
using Files.#readAllLines instead.
second, do not use hard coded indexes, use String#indexOf to find them out. If a substring does not occur in your original string, then the method retunrs -1
, always check for that value and call substring
only when the return value is not -1
(0 or greater).
if(lines.length > 1)
int startIndex = lines[1].indexOf("ert");
if(startIndex != -1)
// do what you want
Btw, there is no point in extracting already known substring from a string
System.out.println(e + "and" + u);
is equivalent to
System.out.println("ertanduio");
Knowing the start and end position of a fixed substring makes only sence if you want to do something with rest of original string, for example removing the substrings.
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
add a comment |
First split your string into lines, you could do this using
String[] lines = readFile.split("[rn]+");
You may want to read the content directly into a List<String>
using Files.#readAllLines instead.
second, do not use hard coded indexes, use String#indexOf to find them out. If a substring does not occur in your original string, then the method retunrs -1
, always check for that value and call substring
only when the return value is not -1
(0 or greater).
if(lines.length > 1)
int startIndex = lines[1].indexOf("ert");
if(startIndex != -1)
// do what you want
Btw, there is no point in extracting already known substring from a string
System.out.println(e + "and" + u);
is equivalent to
System.out.println("ertanduio");
Knowing the start and end position of a fixed substring makes only sence if you want to do something with rest of original string, for example removing the substrings.
First split your string into lines, you could do this using
String[] lines = readFile.split("[rn]+");
You may want to read the content directly into a List<String>
using Files.#readAllLines instead.
second, do not use hard coded indexes, use String#indexOf to find them out. If a substring does not occur in your original string, then the method retunrs -1
, always check for that value and call substring
only when the return value is not -1
(0 or greater).
if(lines.length > 1)
int startIndex = lines[1].indexOf("ert");
if(startIndex != -1)
// do what you want
Btw, there is no point in extracting already known substring from a string
System.out.println(e + "and" + u);
is equivalent to
System.out.println("ertanduio");
Knowing the start and end position of a fixed substring makes only sence if you want to do something with rest of original string, for example removing the substrings.
edited Oct 22 '14 at 14:13
answered Oct 22 '14 at 13:58
A4LA4L
15k43352
15k43352
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
add a comment |
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
readAllLines or stackoverflow.com/questions/326390/… :)
– Menelaos Bakopoulos
Oct 22 '14 at 14:00
add a comment |
You may give this a try:-
Scanner sc=new Scanner(new FileReader(new File(The file path for readFile.txt)));
String st="";
while(sc.hasNext())
st=sc.next();
System.out.println(st.substring(2,5)+" "+"and"+" "+st.substring(6,9));
Check out if it works.
add a comment |
You may give this a try:-
Scanner sc=new Scanner(new FileReader(new File(The file path for readFile.txt)));
String st="";
while(sc.hasNext())
st=sc.next();
System.out.println(st.substring(2,5)+" "+"and"+" "+st.substring(6,9));
Check out if it works.
add a comment |
You may give this a try:-
Scanner sc=new Scanner(new FileReader(new File(The file path for readFile.txt)));
String st="";
while(sc.hasNext())
st=sc.next();
System.out.println(st.substring(2,5)+" "+"and"+" "+st.substring(6,9));
Check out if it works.
You may give this a try:-
Scanner sc=new Scanner(new FileReader(new File(The file path for readFile.txt)));
String st="";
while(sc.hasNext())
st=sc.next();
System.out.println(st.substring(2,5)+" "+"and"+" "+st.substring(6,9));
Check out if it works.
answered Oct 24 '14 at 11:24


Saptak BhattacharyaSaptak Bhattacharya
1066
1066
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f26508762%2fhow-to-extract-words-from-a-string-in-java%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S8,FcM,tLx5fW71Kc,o
It won't work.
What actually happening ?– Suresh Atta
Oct 22 '14 at 13:37
why dont you use regexp for substring? Or why you need this? Maybe if you tell us the problem what you want to solve we can give you a simply solution.
– SüniÚr
Oct 22 '14 at 13:40
Your second line is shorter, 10 characters you try to substring at more. You may use regexps .
– Martin
Oct 22 '14 at 13:41
Why not put each line into it's own string? Why are you combining the entire file's contents into one string?
– barna10
Oct 22 '14 at 13:47
using string spliiter should work
– ha9u63ar
Oct 22 '14 at 14:18